How to Write and Deploy a Smart Contract on Ethereum
You know that Ethereum, with its smart contract functionality, is the perfect platform to bring your vision to life.
But where do you start? How do you write and deploy a smart contract on Ethereum? Don’t worry, I’ve got you covered!
In this blog post, you’d be exploring the process of setting up your development environment to deploy your smart contract on the Ethereum network.
Without having to take your time unnecessarily, kindly delve in right away.
How to Write and Deploy a Smart Contract on Ethereum In 2024
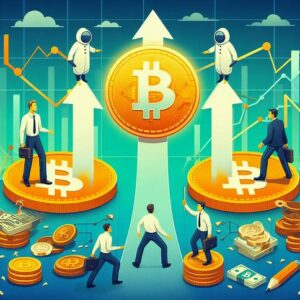
1. You Must First Understand Ethereum and Smart Contracts
Before you delve in too deep, it’s quite essential for you to first understand exactly what Ethereum is and how smart contracts work.
Ethereum is known as a decentralized, open-source blockchain platform that enables developers to build and deploy smart contracts and decentralized applications (dApps).
Unlike Bitcoin, which is primarily used as a digital currency, Ethereum provides a more flexible and programmable blockchain that allows for the creation of complex applications.
Smart contracts are self-executing contracts with the terms of the agreement directly written into code. They are stored and replicated on the Ethereum blockchain, ensuring that the terms of the contract are transparent, immutable, and enforceable without the need for intermediaries. Smart contracts can be used for a wide range of applications, from simple token transfers to complex financial instruments and supply chain management systems.
2. Setting Up Your Development Environment
To start developing smart contracts on Ethereum, you’ll need to set up your development environment. Here’s what you’ll need:
- A computer with an internet connection
- A code editor (e.g., Visual Studio Code, Sublime Text, or Atom)
- Node.js and npm (Node Package Manager) installed on your computer
- An Ethereum wallet (e.g., MetaMask or MyEtherWallet)
- Solidity, the programming language used for writing smart contracts on Ethereum
- Truffle, a development framework for Ethereum that makes it easier to write, test, and deploy smart contracts
Once you have these tools installed, you’re ready to start writing your first smart contract!
3. Writing Your Smart Contract in Solidity
Solidity is the most popular programming language for writing smart contracts on Ethereum.
It is a contract-oriented, high-level language that is influenced by C++, Python, and JavaScript.
Solidity is designed to be easy to learn for developers who are familiar with object-oriented programming.
Here’s an example of a simple smart contract written in Solidity:
solidity
Copy code
pragma solidity ^0.8.0;
contract SimpleStorage {
uint256 private storedData;
function set(uint256 x) public {
storedData = x;
}
function get() public view returns (uint256) {
return storedData;
}
}
This smart contract, called “SimpleStorage,” allows users to store and retrieve a single unsigned integer value.
The set function allows users to store a value, while the get function allows users to retrieve the stored value.
When writing smart contracts in Solidity, kindly note the following as the best practices:
- Using clear and descriptive names for variables and functions
- Validating user inputs to prevent unexpected behavior
- Implementing access control to restrict certain functions to authorized users
- Testing your smart contract thoroughly before deploying it to the Ethereum network
4. Compiling and Testing Your Smart
Contract Once you’ve written your smart contract in Solidity, the next step is to compile it to ensure that there are no syntax errors and that it can be deployed to the Ethereum network. Truffle makes this process easy with its built-in compiler and testing framework.
To compile your smart contract using Truffle, simply navigate to your project directory in the terminal and run the following command:
Copy code
truffle compile
This will compile your smart contract and generate a JSON file containing the compiled bytecode and ABI (Application Binary Interface) for your contract.
Next, you’ll want to test your smart contract to ensure that it behaves as expected.
Truffle provides a testing framework that allows you to write and run tests for your smart contract using JavaScript. Here’s an example of a simple test for the “SimpleStorage” contract:
javascript
Copy code
const SimpleStorage = artifacts.require(“SimpleStorage”);
contract(“SimpleStorage”, accounts => {
it(“should store the value 89.”, async () => {
const simpleStorageInstance = await SimpleStorage.deployed();
// Set value of 89
await simpleStorageInstance.set(89, { from: accounts[0] });
// Get stored value
const storedData = await simpleStorageInstance.get.call();
assert.equal(storedData, 89, “The value 89 was not stored.”);
});
});
This test deploys an instance of the “SimpleStorage” contract, sets a value of 89, and then retrieves the stored value to ensure that it matches the expected value of 89. To run this test, simply run the following command in your terminal:
Copy code
truffle test
5. Deploying Your Smart Contract to the Ethereum Network
Once you’ve compiled and tested your smart contract, you’re ready to deploy it to the Ethereum network.
There are several ways to deploy a smart contract, but the easiest and most common method is to use Truffle.
To deploy your smart contract using Truffle, you’ll first need to create a deployment script.
Here’s an example deployment script for the “SimpleStorage” contract:
javascript
Copy code
const SimpleStorage = artifacts.require(“SimpleStorage”);
module.exports = function(deployer) {
deployer.deploy(SimpleStorage);
};
This script tells Truffle to deploy an instance of the “SimpleStorage” contract to the Ethereum network. To run this script and deploy your contract, simply run the following command in your terminal:
Copy code
truffle migrate
This will compile your smart contract (if it hasn’t already been compiled), deploy it to the Ethereum network, and provide you with the address of your deployed contract.
6. Interacting with Your Deployed Smart Contract
Once your smart contract is deployed on the Ethereum network, you can interact with it using a variety of tools and frameworks.
One popular method is to use web3.js, a JavaScript library that allows you to interact with the Ethereum blockchain from a web application.
Here’s an example of how you can use web3.js to interact with the deployed “SimpleStorage” contract:
javascript
Copy code
const contractAddress = “0x1234…”; // Replace with your contract address
const contractABI = [{“constant”:false,“inputs”:[{“name”:“x”,“type”:“uint256”}],“name”:“set”,“outputs”:[],“payable”:false,“stateMutability”:“nonpayable”,“type”:“function”},{“constant”:true,“inputs”:[],“name”:“get”,“outputs”:[{“name”:“”,“type”:“uint256”}],“payable”:false,“stateMutability”:“view”,“type”:“function”}]; // Replace with your contract ABI
const web3 = new Web3(new Web3.providers.HttpProvider(“https://mainnet.infura.io/v3/YOUR_PROJECT_ID”)); // Replace with your Infura project ID
const contract = new web3.eth.Contract(contractABI, contractAddress);
// Set the value to 42
contract.methods.set(42).send({ from: “0x1234…” }); // Replace with your Ethereum address
// Get the stored value
contract.methods.get().call().then(function(result) {
console.log(result);
});
This code snippet creates a new web3 instance using an Infura endpoint (which allows you to interact with the Ethereum network without running your own node), creates a new contract instance using the contract address and ABI, sets the stored value to 42, and then retrieves the stored value and logs it to the console
Read: Bitcoin vs. Traditional Investments (Everything You Need To Know)
7. Best Practices for Smart Contract
Development Writing and deploying smart contracts on Ethereum can be a complex process, and I’ll implore you to follow best practices for you to ensure that your contracts are secure, efficient, and maintainable.
Here are some important you may have to keep in mind:
- Use well-established and audited libraries and tools whenever possible, rather than writing everything from scratch.
- Keep your contracts simple and modular, with clear separation of concerns between different parts of the contract.
- Use access control mechanisms to restrict access to sensitive functions and data, and to prevent unauthorized access.
- Avoid using external calls to untrusted contracts or sources of data, as this can introduce security vulnerabilities.
- Test your contracts thoroughly, using both automated tests and manual testing, to ensure that they behave as expected and are free of bugs and vulnerabilities.
- Use version control (e.g., Git) to track changes to your contracts over time and to facilitate collaboration with other developers.
- Follow the Solidity style guide and naming conventions to ensure that your code is readable and maintainable.
- Keep up to date with the latest security best practices and vulnerabilities in the Ethereum ecosystem, and update your contracts as needed to address any issues.
By following these best practices, you can help ensure that your smart contracts are secure, efficient, and maintainable over the long term.
Related: How Do Smart Contracts Work on the Ethereum Blockchain?
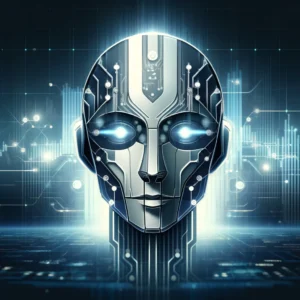
Key Takeaways:
- Ethereum is a decentralized, open-source blockchain platform that enables developers to build and deploy smart contracts and decentralized applications (dApps).
- Smart contracts are self-executing contracts with the terms of the agreement directly written into code, stored and replicated on the Ethereum blockchain.
- To develop smart contracts on Ethereum, you need to set up your development environment with tools like Solidity, Truffle, and an Ethereum wallet.
- Solidity is the most popular programming language for writing smart contracts on Ethereum, designed to be easy to learn for developers familiar with object-oriented programming.
- After writing your smart contract in Solidity, you need to compile it, test it using a testing framework like Truffle, and then deploy it to the Ethereum network.
- Once your smart contract is deployed, you can interact with it using tools and frameworks like web3.js, which allows you to interact with the Ethereum blockchain from a web application.
- Following best practices for smart contract development, such as using well-established libraries, keeping contracts simple and modular, and thoroughly testing contracts, is crucial for ensuring security, efficiency, and maintainability.
- The Ethereum ecosystem offers a wealth of resources for further learning, including official documentation, community-driven Q&A sites, and comprehensive lists of developer tools.
- As the Ethereum ecosystem continues to grow, smart contracts have the potential to disrupt a wide range of industries and create new opportunities for innovation and growth.
- Ethereum developers have the opportunity to be at the forefront of the exciting and rapidly evolving ecosystem of decentralized technology, building applications and infrastructure that will shape the future of the internet and the global economy.
FAQs | Frequently Asked Questions
Here are some helpful frequently asked questions on how to write and deploy a smart contract on Ethereum with answers:
What is the difference between Ethereum and Bitcoin?
While both Ethereum and Bitcoin are decentralized blockchain platforms, Ethereum enables developers to build and deploy smart contracts and decentralized applications (dApps), whereas Bitcoin primarily functions as a digital currency.
Ethereum’s programmable blockchain allows for more complex transactions and agreements to be executed automatically.
Do I need to know programming to write smart contracts on Ethereum?
Yes, writing smart contracts on Ethereum requires knowledge of programming, specifically in Solidity, which is the most popular language for Ethereum smart contract development. Familiarity with object-oriented programming concepts is also beneficial.
What is gas in Ethereum, and why is it important?
In Ethereum, gas refers to the cost required to perform a transaction or execute a smart contract.
Gas is paid in Ether (ETH), the native cryptocurrency of Ethereum. It is important because it incentives miners to process transactions and prevents network spam by requiring a fee for each operation.
Can I test my smart contract without deploying it to the main Ethereum network?
Yes, you can test your smart contract using a local development blockchain like Ganache, which simulates the Ethereum network on your computer.
This allows you to test your contract’s functionality and identify potential issues before deploying it to the main network.
What happens if I deploy a smart contract with bugs or vulnerabilities?
Once a smart contract is deployed to the Ethereum network, it cannot be modified or updated.
This is why thorough testing and auditing of smart contracts are crucial before deployment.
If a contract contains bugs or vulnerabilities, it may result in unintended behavior, financial losses, or even theft of funds.
Conclusion
To wrap it up, writing and deploying smart contracts on Ethereum can seem like a daunting task at first, but with the right tools and best practices, it’s a process that anyone can learn and master.
By understanding the basics of Ethereum and smart contracts, setting up your development environment, writing your contracts in Solidity, compiling and testing your contracts, deploying them to the Ethereum network, and interacting with them using web3.js or other tools, you can build powerful and innovative decentralized applications that have the potential to make changes in the industries and change the world.